Vue Js Get nth item/element of array:To get the nth item or element of an array in Vue.js, you can use array indexing. In JavaScript, arrays are zero-indexed, meaning the first item has an index of 0. To access the nth item, you can use the index n-1. For example, if you have an array called “myArray” and you want to get the third item, you can use “myArray[2]”. This will return the item at index 2, which is the third item in the array.
How can I retrieve the nth item/element of an array in Vue js?
The given Vue.js code snippet demonstrates how to get the nth item/element of an array using Vue.js. The Vue instance is created and bound to the HTML element with the ID “app”. The data object contains an array called “myArray” with four elements.
Within the Vue instance, there is a method called “getNthItem” that takes an index parameter “n”. If the index is within the valid range of the array, it returns the corresponding item from the array. Otherwise, it returns the string “Invalid index”.
In the HTML template, the result of calling the “getNthItem” method with the index 2 is displayed using the double curly braces notation ({{ }}).
Vue Js Get nth item/element of array Example
<div id="app">
<p>The nth item of the array is: {{ getNthItem(2) }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myArray: ['Vue', 'React', 'Angular', 'Node']
};
},
methods: {
getNthItem(n) {
if (n >= 0 && n < this.myArray.length) {
return this.myArray[n];
} else {
return 'Invalid index';
}
}
}
});
</script>
Output of Vue Js Get nth item/element of array
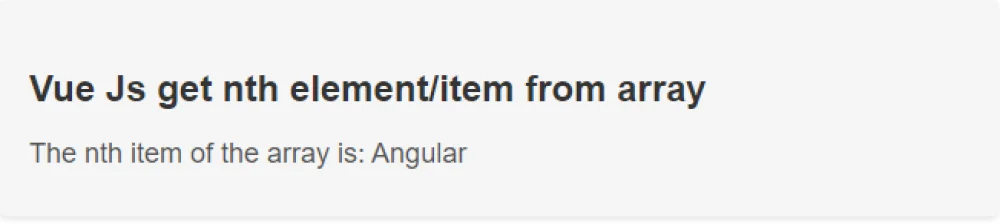